Using Mongoose With AWS Lambda
AWS Lambda is a popular service for running arbitrary functions without managing individual servers. Using Mongoose in your AWS Lambda functions is easy. Here's a sample function that connects to a MongoDB instance and finds a single document:
const mongoose = require('mongoose');
let conn = null;
const uri = 'YOUR CONNECTION STRING HERE';
exports.handler = async function(event, context) {
// Make sure to add this so you can re-use `conn` between function calls.
// See https://www.mongodb.com/blog/post/serverless-development-with-nodejs-aws-lambda-mongodb-atlas
context.callbackWaitsForEmptyEventLoop = false;
// Because `conn` is in the global scope, Lambda may retain it between
// function calls thanks to `callbackWaitsForEmptyEventLoop`.
// This means your Lambda function doesn't have to go through the
// potentially expensive process of connecting to MongoDB every time.
if (conn == null) {
conn = mongoose.createConnection(uri, {
// Buffering means mongoose will queue up operations if it gets
// disconnected from MongoDB and send them when it reconnects.
// With serverless, better to fail fast if not connected.
bufferCommands: false, // Disable mongoose buffering
bufferMaxEntries: 0 // and MongoDB driver buffering
});
// `await`ing connection after assigning to the `conn` variable
// to avoid multiple function calls creating new connections
await conn;
conn.model('Test', new mongoose.Schema({ name: String }));
}
const M = conn.model('Test');
const doc = await M.findOne();
console.log(doc);
return doc;
};
To import this function into Lambda, go the AWS Lambda console and click "Create Function".
Create a function called "mongoose-test" with the below settings:
Copy the source code into a file called lambda.js
. Then run npm install mongoose co
.
Finally, run zip -r mongoose-test.zip node_modules/ lambda.js
to create a
zip that you can upload to Lambda using the "Upload a Zip File" option under "Function code".
Make sure you also change the "Handler" input to lambda.handler
to match the lambda.js
file's handler
function.
Next, click the "Save" button and then the "Test" button. The "Test" button will ask you to create a new test event, just create one because your inputs don't matter for this example. Then, hit "Test" again to actually run your function:
If your MongoDB database goes down in between function calls, you may see the below error message:
cannot find account after reload: could not find config for <hostname>
Lambda's JavaScript framework recently added support for async/await as long as you're using Node 8.x, so make sure you're not using Node.js 6.x.
Want to learn how to check whether your favorite JavaScript frameworks, like Express or React, work with async/await? Spoiler alert: neither Express nor React support async/await. Chapter 4 of Mastering Async/Await explains the basic principles for determining whether a framework supports async/await. Get your copy!
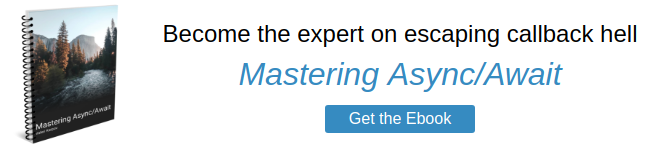